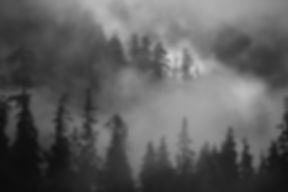
What are Intelligent systems?
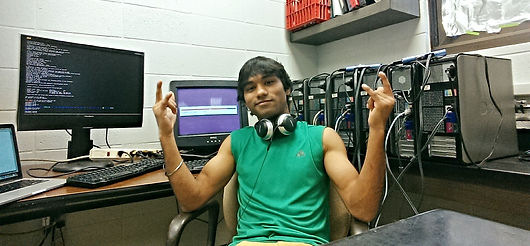
If the system can evolve on its own...then its definitly kinda intelligent.
Now the next question is...why do we need intelligent systems? I am not sure if I have the right answer to this question. Maybe because I am too lazy? God knows.
II need intelligent systems because they make me look cool and they help me to analyze datasets from a multidimensional perspective.
Computers are fascinating objects and its worth understanding them. Its always good to start from the basic concepts of number theorums and sequences. So, The first task here is to detect if the given set of numbers are rational or irrational. Now this might take a while if we try doing this for a set of millions of real numbers and by using our highly intellectual and complicated brain network. The alternate way to ask computers nicely. And the question here is, how do we ask computers nicely and what instructions shall we pass to get this thing done...So, lets define rational numbers first.
The rational numbers can be formally defined as the equivalence classes of the quotient set (Z × (Z \ {0})) / ~, where the cartesian product Z × (Z \ {0}) is the set of allordered pairs (m,n) where m and n are integers, n is not 0 (n ≠0), and "~" is the equivalence relation defined by (m1,n1) ~ (m2,n2) if, and only if, m1n2 − m2n1 = 0.
I know its complicated. In simple sentence, rational numbers are represented as a/b where a and b are co-prime and a and b are integers, otherwise its an irrational number. Thomae's function uses this information to create a plot of rational numbers, which looks discontinous. According to Thomae's method:
Here is a real value function of real x. If x ie. p/q is a rational number, Thomae's function return 1/q and otherwise it returns zero. So, our job is to write a program that mimicks Thomae's function. Below is my Thomae's function python script:
import matplotlib.pyplot as plt
def thomae_rational_points(values):
return [(float(1)/float(x.split('/')[1]), float(x.split('/')[0])/float(x.split('/')[1])) for x in values]
def rational_numbers(max_denominator):
fract = list()
i=2
while i <=max_denominator:
j=1
while j <= i-1:
fract.append(str(j) + '/' + str(i))
j = j + 1
i = i + 1
return fract
max_denominator = 500
points=(thomae_rational_points(rational_numbers(max_denominator)))
point1 = list(); point2 = list()
for (x,y) in points:
point1.append(x); point2.append(y)
plt.plot(point2, point1, 'ro')
plt.savefig('fig.png')
Figure on the right side is the output obtained from the above program. Points are plotted between p/q and 1/q for rationals between 0 to 1 with the highest denominator of 500 (Since we cant do it for infinite numbers). As we can see here, the points are not equidistant from each other. There is a clear discontinuity between the points, which is a property of rational numbers. Points generated by irrational numbers are continous. So, if we get a set of discontinous points for a set of given numbers, it tells us that the set contains rational numbers in it.
Now this was a basic method to differentiate between rational and irrational numbers. Lets use this to make our machine intelligent. Lets consider a situation where two new half-muggles of Hogwarts A and B has five different set of reals.
A Set1 Set2 Set3 Set4 Set5
B Set6 Set7 Set8 Set9 Set10
Now let us assume that their selection in Gryffindor House depends on the composition of rationals in these sets collected from the half-muggles and in this case we already know that A is in Gryffindor and B is not. So, now lets create a training dataset. First step will be to calculate the composition of rational numbers in each sets.
To do this we will use Thomae's function. We have already seen that Thomae's function can clearly distinguish between rationals and irrational numbers. So, by applying Thomae's function to each set of reals, we can easily calculate the composition of rational numbers in each set.
Pseudocode to do this is given below
groups = ['A', 'B', 'C']
for i, individual in enumerate(groups):
for j, sets in enumerate(individual):
rationals = [x for x in sets if Thomaes(x) == 'Rationals']
groups[i][j] = float(len(rationals))/len(sets)
Gives an output
A 0.42 0.58 0.11 0.23 0.89
B 0.33 0.89 0.47 0.31 0.54
So, now we have training data with composition of rationals in 5 different set groups for individuals in Gryffindor (A) (positive) and individuals not in Gryffindor (B) (negative). Now lets train our machine using support vector algorithm. No Wait!!! Let me introduce you to some basic concepts of support vector classification method and methods to do it in python.
Support Vector Classification
In machine learning, support vector machines (SVMs, also support vector networks) are supervised learning models with associated learning algorithms that analyze data and recognize patterns, used for classification and regression analysis.....bla bla bla bla blaaaaaaaaa.......hold on....let me make it simple. Follow these steps:
Step 1. Let us assume that you have size of Eyes, Nose and Head of 10 European and 10 American girls.
Step 2. Lets pick the number 0 for European and 1 for American girls.
Step 3. Now when you feed this dataset as a training data in SVM, it creates two profiles, one each for European (negative, since its set to 0) and American (posotive, since its set to 1). Each of these two profile contains vectors used for new data classification.
Now these profiles can be used to check if any new set of Nose, Eyes and Head size belong to a European or an American girl. In short, SVM can be used for classification of a new dataset into postive or negative category.
You got it..right?? Now lets jump into the Gryffindor's issue.
To solve Gryffindor's issue, here we need a tool or a package that can perform SVM classification without too much of programming effort. You definitly don't want me to go into algorithmic details and hardcore machine learning programming at this stage. So lets do it in python scikit-learn python module.
Open terminal -> type python and enter ->
>>> from sklearn import svm
>>> X = [[0.42, 0.58, 0.11, 0.23, 0.89], [0.33, 0.89, 0.47, 0.31, 0.54]]
>>> y = [1, 0]
>>> clf = svm.SVC()
>>> clf.fit(X, y)
SVC(C=1.0, cache_size=200, class_weight=None, coef0=0.0, degree=3, gamma=0.0,
kernel='rbf', max_iter=-1, probability=False, random_state=None,
shrinking=True, tol=0.001, verbose=False)
Now you have your model ready for classification.
Now, a pretty girl walks into you and talks in some nice british accent and she is not Emma Watson. What do we do now. You gotta be kidding me!! You cant just let her go. Not if you are a die-hard Harry Potter fan. Well I am...so I am gonna test if she fits into Gryffindor category or not. So what do we do next. Lets go and talk to her and try to collect five set of real numbers.
Let Z" be the set which contains 5 set of reals collected from the pretty girl with nice british accent. Calculate the composition of rationals in each of these 5 sets and store it in set Z.
Z = [0.32, 0.81, 0.40, 0.27, 0.54]
now go back to your terminal and type
>>> clf.predict([[0.32, 0.81, 0.40, 0.27, 0.54]])
array([0])
Oooooopss....array([0]) means she does not belong to Gryffindor. May be Slytherin, but we aint interested anymore. I would have asked her out on a date if the answer was array([1]). Anyhoooooooo....you got the concept right....


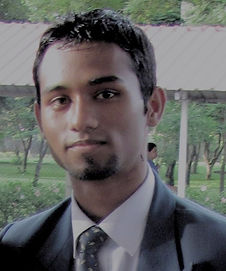
Ambuj Kumar
PhD Student, Bioinformatics
Iowa State University
Editorial Board Member
International Journal of Pharmacy and Pharmaceutical Sciences
Trends Journal of Sciences Research
Journal Refree
International Journal of Computing and Digital Systems
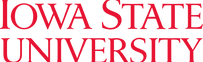

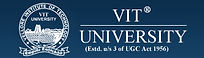